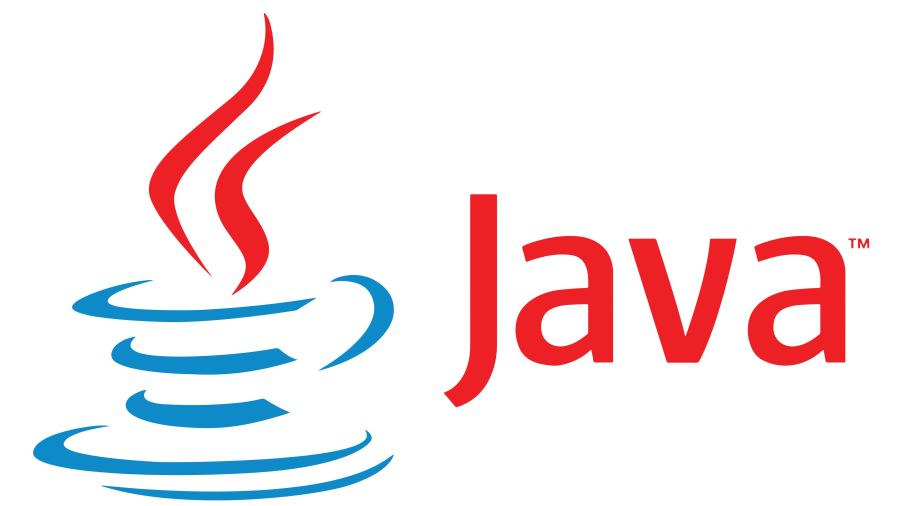
Hashmap은 순서를 보장하지 않는다.
Hashmap을 정렬하는 방법을 알아보자.
트리 맵 사용하기
Treemap은 SortedMap 인터페이스를 상속받는 클래스다.
TreeMap에 Comparator를 생략하면 기본 Comparator가 동작한다. Comparator에서 문자열을 비교할때, compareTo()메소드가 실행됨.
Map<String, Integer> map = new HashMap<>();
map.put("John", 34);
map.put("Jane", 26);
map.put("Tom", 27);
map.put("Bill", 29);
Map<String, Integer> sortedMap = new TreeMap<>(map);
System.out.println(sortedMap);
직접 new Comparator<T>()를 전다하여 정렬 로직을 구현할 수도 있다.
compare 메소드를 재정의 해서 해당 key의 길이로 정렬하고 길이가 동일하면 사전순으로 정렬하는 로직이다.
Map<String, Integer> map = new HashMap<>();
map.put("John", 34);
map.put("Jane", 26);
map.put("Tom", 27);
map.put("Bill", 29);
Map<String, Integer> sortedMap = new TreeMap<>(new Comparator<String>() {
@Override
public int compare(String o1, String o2) {
int lengthDifference = o1.length() - o2.length();
if (lengthDifference != 0) return lengthDifference;
return o1.compareTo(o2);
}
});
sortedMap.putAll(map);
System.out.println(sortedMap);
ArrayList 사용하기
- Sort by Key
해당 Key로 ArrayList를 만들어 Collections.sort를 이용해 정렬하는 방법이다.
List<String> employeeByKey = new ArrayList<>(map.keySet());
Collections.sort(employeeByKey);
- Sort by Value
List<Employee> employeeById = new ArrayList<>(map.values());
Collections.sort(employeeById);
해당 방법으로 정렬을 할 수 있는 이유는 Employee가 Comparable 인터페이스를 구현하고 있기 때문이다!!
public class Employee implements Comparable<Employee> {
private Long id;
private String name;
// constructor, getters, setters
// override equals and hashCode
@Override
public int compareTo(Employee employee) {
return (int)(this.id - employee.getId());
}
}
TreeSet 이용하기
- Sort by Key
SortedSet<String> keySet = new TreeSet<>(map.keySet());
- Sort by Value
SortedSet<Employee> values = new TreeSet<>(map.values());
람다와 스트림 이용
- Sort by Key
map.entrySet()
.stream()
.sorted(Map.Entry.<String, Employee>comparingByKey())
.forEach(System.out::println);
- Sort by Value
map.entrySet()
.stream()
.sorted(Map.Entry.comparingByValue())
.forEach(System.out::println);
- 새로운 맵으로 결과를 받는 방법 (LinkedHashMap)
LinkedHashMap은 삽입순서를 유지하는 클래스이다. LinkedHashMap클래스는 이중 연결리스트 방식으로 데이터 요소를 접근하는데 용이함.
Map<String, Employee> result = map.entrySet()
.stream()
.sorted(Map.Entry.comparingByValue())
.collect(Collectors.toMap(
Map.Entry::getKey,
Map.Entry::getValue,
(a, b) -> { throw new AssertionError(); },
LinkedHashMap::new
));
'Language > Java' 카테고리의 다른 글
[Java] 배열을 List로 변환하기, List를 배열로 변환하기, convert int array to arrayList, convert list to int array (0) | 2023.02.03 |
---|---|
[Java] Iterator 정리 / Map , ArrayList, Set에서의 iterate 사용법 (0) | 2023.02.02 |
[Java] 람다 표현식 / 함수형 인터페이스 (@FunctionalInterface) (0) | 2023.01.31 |
[Java] 익명함수 (익명 자식 객체, 익명 구현 객체) (0) | 2023.01.31 |
[Java] 오버로딩 & 오버라이딩 Overloading / Overriding (0) | 2023.01.31 |
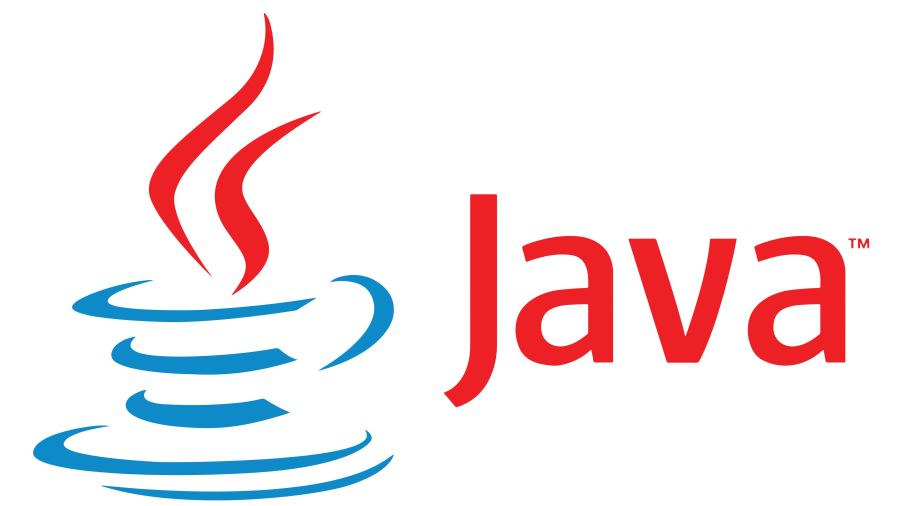
Hashmap은 순서를 보장하지 않는다.
Hashmap을 정렬하는 방법을 알아보자.
트리 맵 사용하기
Treemap은 SortedMap 인터페이스를 상속받는 클래스다.
TreeMap에 Comparator를 생략하면 기본 Comparator가 동작한다. Comparator에서 문자열을 비교할때, compareTo()메소드가 실행됨.
Map<String, Integer> map = new HashMap<>();
map.put("John", 34);
map.put("Jane", 26);
map.put("Tom", 27);
map.put("Bill", 29);
Map<String, Integer> sortedMap = new TreeMap<>(map);
System.out.println(sortedMap);
직접 new Comparator<T>()를 전다하여 정렬 로직을 구현할 수도 있다.
compare 메소드를 재정의 해서 해당 key의 길이로 정렬하고 길이가 동일하면 사전순으로 정렬하는 로직이다.
Map<String, Integer> map = new HashMap<>();
map.put("John", 34);
map.put("Jane", 26);
map.put("Tom", 27);
map.put("Bill", 29);
Map<String, Integer> sortedMap = new TreeMap<>(new Comparator<String>() {
@Override
public int compare(String o1, String o2) {
int lengthDifference = o1.length() - o2.length();
if (lengthDifference != 0) return lengthDifference;
return o1.compareTo(o2);
}
});
sortedMap.putAll(map);
System.out.println(sortedMap);
ArrayList 사용하기
- Sort by Key
해당 Key로 ArrayList를 만들어 Collections.sort를 이용해 정렬하는 방법이다.
List<String> employeeByKey = new ArrayList<>(map.keySet());
Collections.sort(employeeByKey);
- Sort by Value
List<Employee> employeeById = new ArrayList<>(map.values());
Collections.sort(employeeById);
해당 방법으로 정렬을 할 수 있는 이유는 Employee가 Comparable 인터페이스를 구현하고 있기 때문이다!!
public class Employee implements Comparable<Employee> {
private Long id;
private String name;
// constructor, getters, setters
// override equals and hashCode
@Override
public int compareTo(Employee employee) {
return (int)(this.id - employee.getId());
}
}
TreeSet 이용하기
- Sort by Key
SortedSet<String> keySet = new TreeSet<>(map.keySet());
- Sort by Value
SortedSet<Employee> values = new TreeSet<>(map.values());
람다와 스트림 이용
- Sort by Key
map.entrySet()
.stream()
.sorted(Map.Entry.<String, Employee>comparingByKey())
.forEach(System.out::println);
- Sort by Value
map.entrySet()
.stream()
.sorted(Map.Entry.comparingByValue())
.forEach(System.out::println);
- 새로운 맵으로 결과를 받는 방법 (LinkedHashMap)
LinkedHashMap은 삽입순서를 유지하는 클래스이다. LinkedHashMap클래스는 이중 연결리스트 방식으로 데이터 요소를 접근하는데 용이함.
Map<String, Employee> result = map.entrySet()
.stream()
.sorted(Map.Entry.comparingByValue())
.collect(Collectors.toMap(
Map.Entry::getKey,
Map.Entry::getValue,
(a, b) -> { throw new AssertionError(); },
LinkedHashMap::new
));
'Language > Java' 카테고리의 다른 글
[Java] 배열을 List로 변환하기, List를 배열로 변환하기, convert int array to arrayList, convert list to int array (0) | 2023.02.03 |
---|---|
[Java] Iterator 정리 / Map , ArrayList, Set에서의 iterate 사용법 (0) | 2023.02.02 |
[Java] 람다 표현식 / 함수형 인터페이스 (@FunctionalInterface) (0) | 2023.01.31 |
[Java] 익명함수 (익명 자식 객체, 익명 구현 객체) (0) | 2023.01.31 |
[Java] 오버로딩 & 오버라이딩 Overloading / Overriding (0) | 2023.01.31 |